We have all been told that blockchain data is public and available for everyone to see and that it’s super easy to query. But… have you tried doing this? How do we know the wallet balance of an ERC-20 without a block explorer? How do I see the transaction history of a wallet? It’s all easier said than done.
In this article, you will learn how the Sequence Indexer helps you solve all these problems (and many more) with simple API calls. We will use a small demo app built with Next.js, React, and typescript. If you are not familiar with the stack, don’t worry! All you need is a basic understanding of using APIs, and you’ll be able to follow along.
Learning to take advantage of an Indexer will allow you to build dapps much faster and more reliably.
What querying the blockchain looks like WITHOUT Sequence indexer?
To query blockchain data, you would need access to a node. Nodes are interconnected entities that participate in a blockchain by validating blocks and storing data. You can run your own node (which is resource intensive and requires extra steps) or use a node provider (Sequence Node Gateway is a great option).
Then you would be able to query some data directly, but not all nodes retain all the history of a blockchain. So, you would have to constantly listen to events to keep up to date with the new blocks or query a full node. All in all, indexing the blockchain is crucial but hard to do and is pretty messy–hence why most people opt to use an indexer instead!
What querying the blockchain looks like WITH Sequence Indexer?
The Sequence Indexer handles all the messy, complex work of querying nodes and listening to events for you. Regardless of which language you’ve built your app in, you just need to make an API call to get the data you need. It’s that simple.
Before we jump into the tutorial, you can always familiarize yourself with the official Sequence Indexer documentation, but it shouldn’t be necessary to follow the tutorial.
We have prepared a small demo app with a starter code, and the end results in our repo for you to clone and play around with. We’ll be using the code from there to show how some endpoints work, but you don’t need to clone it if you don’t want to. Let’s jump right in:
Cloning the demo app
Note — This was published in February 2023. While all efforts will be made to keep the tutorial up-to-date, always refer to the Sequence Docs for the most up-to-date information.
Clone this repository into any folder you like and then go into the ‘code-samples/sequence-indexer’ folder. Here you will find a demo app made with Next.js, React, and typescript that you can play around with. If you want the starting coding app without any API calls, you can switch to the ‘starter-code’ branch and do it all on your own!
git clone git@github.com:horizon-games/code-samples.gitcd code-samples/sequence-indexer
Installing Sequence dependency & running the app
To install the dependencies and use the Sequence Indexer, you would need to run this command:
npm install 0xsequence ethers
Sequence uses the ethers.js library to work so we need that as well. But since our demo app package.json already has these dependencies, you can just run:
#Run these commands after cloning the reponpm installnpm run dev
The app will now start running and going to http://localhost:3000/ on your browser will take you to our app!
Query token balances for a specific wallet
Let’s use the Tokens API to get the token balances of any wallet we want. To do this, I created the getWalletBalances() function to call the Tokens API and update our balances React state.
As you can see in the code below, we first instantiate the indexer using the network we want. In this case, we are using Ethereum mainnet, but you can check all available networks here. Then you can use the indexer to call .getTokenBalances() and pass the input object like this:
const [balances, setBalances] = React.useState<TokenBalance[]>([]);// Gets the wallet token and updates the token balance stateconst getWalletBalances = async () => {// Instantiate the indexer in the selected networkconst indexer = new SequenceIndexerClient('https://mainnet-indexer.sequence.app');// Choose any wallet you wantconst walletAddress = '0xabc..';// Call Tokens API to get all token balancesconst tokenBalances = await indexer.getTokenBalances({accountAddress: walletAddress,includeMetadata: true});setBalances(tokenBalances.balances);}
The input object for getTokenBalances() looks like below. If the property has a ‘?’, it’s optional. Depending on the properties you use, you will get different information. For example, adding a specific contract address will give you the balance in the wallet selected for that specific token. The “page” property is added to allow us to iterate over the result in case the wallet we are looking at has several different tokens.
// Arguments object for getTokenBalancesexport interface GetTokenBalancesArgs {accountAddress?: string;contractAddress?: string;includeMetadata?: boolean;page?: Page;}
Ok, those are the possible inputs, but what does the output look like?
// Example output with only one token in the wallet.{"page": {"page": 2,"pageSize": 1000,"more": false},"balances": [{"contractAddress": "0x9813037ee2218799597d83d4a5b6f3b6778218d9","contractType": "ERC20","accountAddress": "0x645ccbca5b0d493a205112663381f3dc69ea4e94","tokenID": "0","balance": "85215104162718583410","updateId": 0,"chainId": 1,"contractInfo": {"chainId": 1,"address": "0x9813037ee2218799597d83d4a5b6f3b6778218d9","name": "Bone ShibaSwap","type": "UNKNOWN","symbol": "BONE","decimals": 18,"logoURI": "https://assets.coingecko.com/coins/images/16916/thumb/bone_icon.png?1625625505","deployed": false,"bytecodeHash": "","extensions": {"link": "","description": "","ogImage": "","originChainId": 0,"originAddress": ""}}},]}
In the response object, you get the contract address, the balance, decimals to format the balance, the logo URI, and a lot more information. One cool thing I want to point out is that Sequence Indexer will tell you the contractType (ERC20, ERC721, ERC1155), so you can use this info to improve your users’ experience by doing things like showcasing NFT artwork within your dapp.
Query native token balance for a specific wallet
Now I would like to show you the Native Network Balance API. With this endpoint, you can ask for each network’s native balance. This means you will get the ETH balance on Ethereum mainnet, Matic on Polygon, BNB on BSC chain, etc.
To call the .getEtherBalance() method is really easy since we already have the indexer instantiated! I will be doing it all in the same function since I want to make only one call to load token balances and the native balance of a wallet at the same time.
The inputs for this one are very simple, only an object with accountAddress property. In return, you will get an object, and to access the balance in wei, we need to do the following:
// Gets the wallet token and updates the token balance stateconst getWalletBalances = async () => {// Instantiate the indexer in the selected networkconst indexer = new SequenceIndexerClient('https://mainnet-indexer.sequence.app');// Choose any wallet you wantconst walletAddress = '0xabc..';// Call Tokens API to get all token balancesconst tokenBalances = await indexer.getTokenBalances({accountAddress: walletAddress,includeMetadata: true});// Call native balance API to get native balanceconst nativeBalance = await indexer.getEtherBalance({accountAddress: walletAddress,});setBalances(tokenBalances.balances);// We have to access .balance.balanceWei to get the balancesetNativeBalance(nativeBalance.balance.balanceWei);}
That’s all there is to it! But our native balance is now in wei (which is the smallest denomination of ETH, 1 ETH = 1⁰¹⁸ wei). To show the balance in ETH format, we need to do a little formatting and we’ll use ethers.js utils to help us out.
If you copy and paste the code below, it should work to return the wei number in ETH. Basically, we turn our balance in wei to a BigNumber and then use that number as a parameter for the ethers utils to format it into ETH.
ethers.utils.formatEther(BigNumber.from(nativeBalance.balance.balanceWei))
Using the tokens balance API and the native balance one, we get all the information to build a very basic but cool wallet explorer like this:
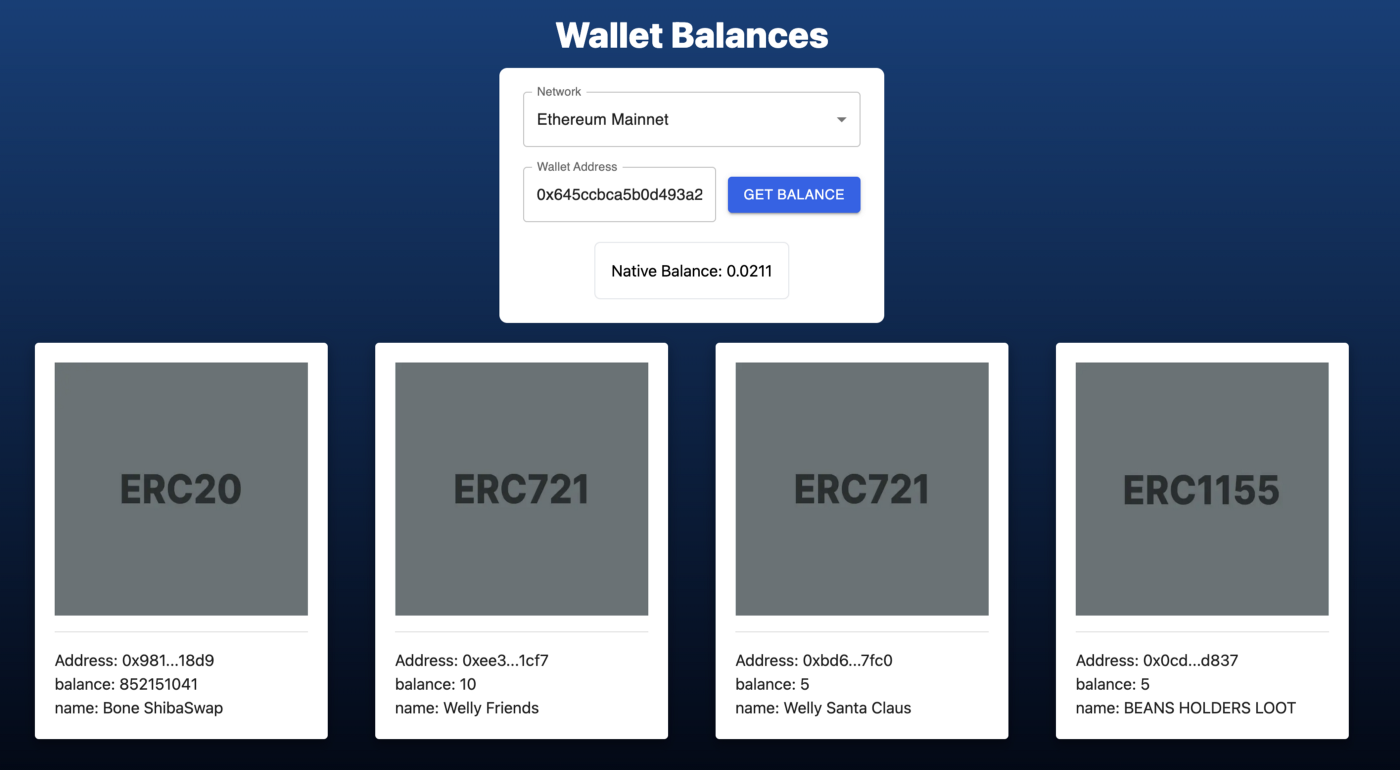
Other useful endpoints and example uses
We went over only two API endpoints that the Sequence Indexer has to offer, but there are a lot more and each is more interesting than the previous one. You can check all of them in the documentation, but here is a brief summary:
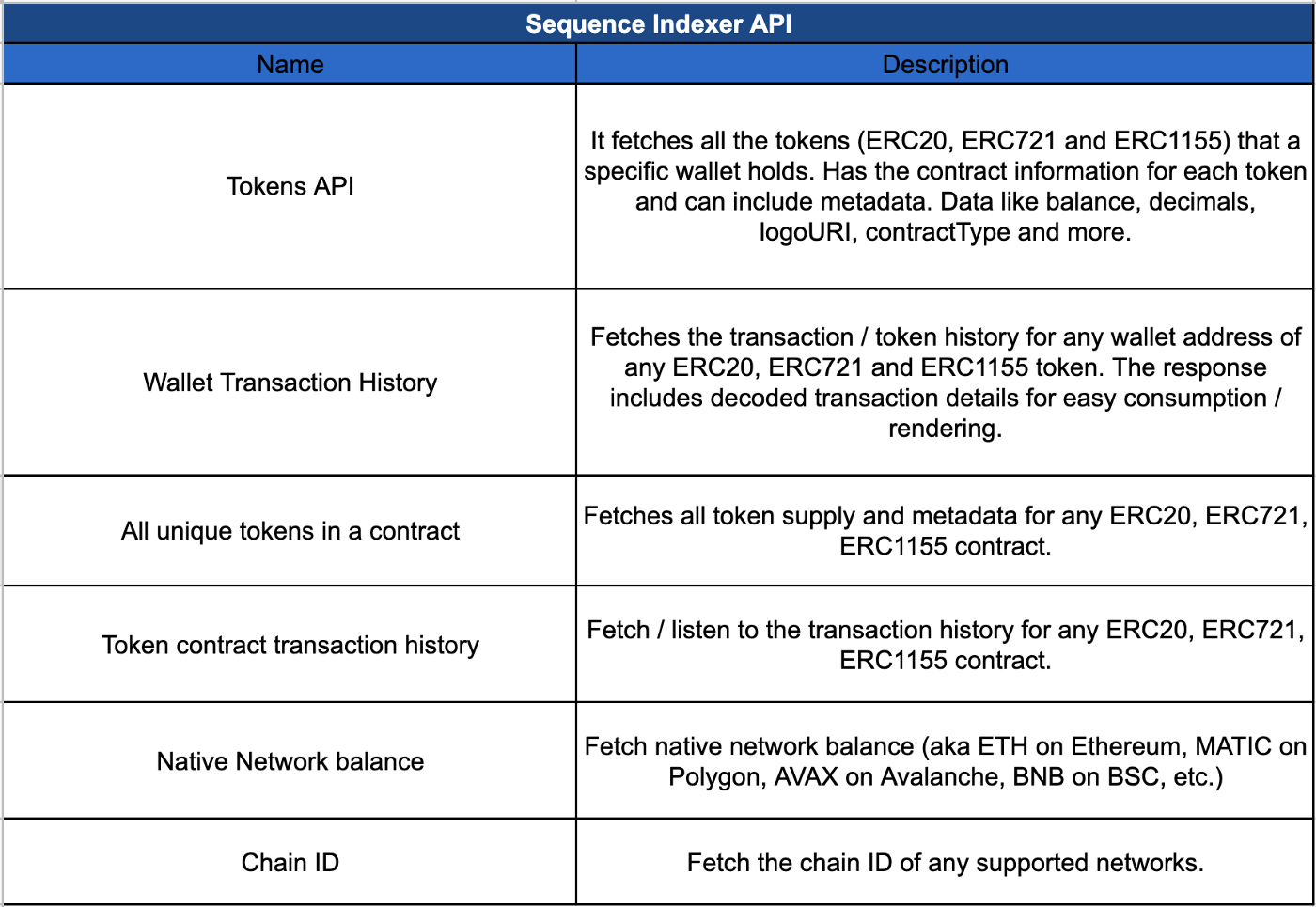
This tutorial serves as an introduction to the features and functionality of the Sequence Indexer API. Whether you already know how to index the blockchain or are just getting started, this API offers a dev-friendly and efficient solution for your blockchain data needs. With its robust set of features (like using several node providers to ensure 99.99% uptime), you’ll be well-equipped to build any dapp that requires blockchain data.
So give it a try, and see the results for yourself! If you have any questions, please do get in touch. We also invite you to join our friendly and resourceful community on Discord 🙂
For a video walkthrough on how to use the Sequence Indexer for your web3 game or dapp, click the link below:
Build, scale, and unify your blockchain ecosystem with Sequence — your all-in-one open-source development platform for chains, games, and apps. One integration gives developers everything they need to create seamless, scalable, and engaging experiences. No more stitching together multiple solutions — just a single, powerful platform that accelerates adoption, enhances user experiences, and drives network effects. Whether you’re launching a new chain, building the next big game, or shipping a breakthrough app, Sequence makes web3 development easy, efficient, and future-proof. Powering the EVM ecosystem of blockchains, thousands of developers, and millions of users, Sequence is backed by leading investors, including Take-Two Interactive, Ubisoft, Xsolla, and Coinbase. https://sequence.xyz/
---
Have any suggestions on how to improve Sequence development platform? Request a new feature here!
Written by
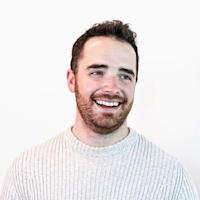
Robert Guenette
Product Marketing DirectorRelated posts